Can Decorators Transform Your Space? Exploring the Art of Interior Design
Can Decorator? Unveiling the Power of Design in Everyday Spaces
In a world where first impressions matter, the art of decoration transcends mere aesthetics; it becomes a powerful tool for expression and transformation. Whether you’re stepping into a cozy café, a bustling office, or the comfort of your own home, the environment speaks volumes about the values and emotions of those who inhabit it. But what if we told you that the essence of decoration goes beyond just choosing colors and furniture? Enter the realm of decorators—professionals who possess the unique ability to weave together elements of design, functionality, and personal style into cohesive spaces that inspire and uplift.
The question “Can Decorator?” invites us to explore the multifaceted role of decorators in shaping our surroundings. These skilled individuals not only curate visual appeal but also consider the psychological impact of design choices, ensuring that each space resonates with its intended purpose. From understanding spatial dynamics to selecting materials that evoke specific feelings, decorators harness a blend of creativity and technical knowledge to create environments that enhance our daily lives.
As we delve deeper into the world of decoration, we will uncover the nuances of this profession, the essential skills required, and the transformative effects that thoughtful design can have on our well-being. Join us on this journey to discover
Understanding Decorators
Decorators in programming are a powerful design pattern that allows behavior to be added to individual functions or methods without modifying their structure. They are commonly used in Python, providing a clean and expressive way to extend the functionality of existing code.
A decorator is essentially a function that takes another function as an argument and extends its behavior without explicitly modifying it. This is particularly useful for scenarios such as logging, access control, and instrumentation.
Key characteristics of decorators include:
- Higher-order functions: Decorators are higher-order functions, which means they can take a function as an argument and return a new function.
- Closure: Decorators often utilize closures, allowing them to maintain state and encapsulate behavior.
- Stacking: Multiple decorators can be applied to a single function, stacking their behaviors.
How Decorators Work
To understand how decorators function, consider the following structure:
- A simple function is defined.
- A decorator function is defined that takes the original function as an argument.
- The decorator adds functionality and returns a new function.
For example:
“`python
def my_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
@my_decorator
def say_hello():
print(“Hello!”)
say_hello()
“`
In this code snippet, the `my_decorator` function wraps the `say_hello` function, allowing additional behavior to be executed before and after the original function call.
Common Use Cases for Decorators
Decorators are used in various scenarios. Here are some common use cases:
- Logging: Automatically log function calls and their parameters.
- Authorization: Check if a user has permission to execute a function.
- Caching: Store results of expensive function calls and return the cached result when the same inputs occur again.
- Timing: Measure the execution time of functions for performance analysis.
Use Case | Description |
---|---|
Logging | Records function execution details for debugging. |
Authorization | Ensures user has the right permissions before executing a function. |
Caching | Improves performance by storing results of expensive calls. |
Timing | Tracks how long a function takes to execute. |
Implementing Custom Decorators
Creating custom decorators can enhance code flexibility and maintainability. Here’s a step-by-step guide to implementing a simple decorator:
- Define the decorator function.
- Inside it, define a wrapper function that contains the logic you want to add.
- Call the original function within the wrapper.
- Return the wrapper function.
For example, if you wanted to create a decorator that repeats the execution of a function, you could do the following:
“`python
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(3)
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
“`
This decorator, `repeat`, allows you to specify how many times the `greet` function should be called, showcasing the flexibility that decorators provide in Python programming.
Understanding Decorators in Python
Decorators in Python provide a powerful and flexible way to modify the behavior of functions or methods. They are often used to add functionality, such as logging, access control, or memoization, without altering the original code structure.
What is a Decorator?
A decorator is essentially a callable (often a function) that takes another function as an argument and extends or alters its behavior. The decorated function is wrapped inside the decorator function, allowing for enhanced functionality.
Basic Syntax of a Decorator
To define a simple decorator, you can use the following syntax:
“`python
def my_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
“`
Applying a Decorator
To apply the decorator to a function, you can use the `@decorator_name` syntax:
“`python
@my_decorator
def say_hello():
print(“Hello!”)
“`
When `say_hello()` is called, it will produce:
“`
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
“`
Common Use Cases
- Logging: Automatically log function calls for debugging purposes.
- Authorization: Check user permissions before executing a function.
- Caching: Store results of expensive function calls to improve performance.
- Timing: Measure the execution time of a function.
Multiple Decorators
You can stack decorators on a single function. The decorators are applied in the order they are listed, from the closest to the function outward.
“`python
@decorator_one
@decorator_two
def my_function():
pass
“`
Decorators with Arguments
Sometimes decorators need to accept arguments. This requires an additional layer of nesting. Here’s how to create a decorator that takes arguments:
“`python
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
“`
Key Points to Remember
- Decorators enhance the behavior of functions or methods.
- They can be applied to any callable object.
- You can create decorators that accept arguments.
- They maintain the metadata of the original function using the `functools.wraps` decorator.
Example: Timing a Function
Here’s a practical example of a decorator that measures the execution time of a function:
“`python
import time
def timer(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f”Function {func.__name__} took {end_time – start_time:.4f} seconds to execute.”)
return result
return wrapper
@timer
def some_function():
time.sleep(2)
some_function()
“`
This example will output the time taken to execute `some_function`.
Conclusion
Python decorators are a versatile feature that can significantly enhance code functionality while promoting clean and maintainable code structures. Understanding how to implement and utilize decorators effectively can greatly improve the efficiency and readability of your Python code.
Exploring the Potential of Decorators in Programming
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “Decorators provide a powerful way to enhance the functionality of functions and methods in a clean and readable manner. They allow developers to adhere to the DRY principle by abstracting repetitive code into reusable components.”
Michael Chen (Lead Developer, CodeCraft Solutions). “The use of decorators can significantly improve code organization and readability. By applying decorators, developers can separate concerns in their codebase, making it easier to maintain and extend functionalities without altering the original code.”
Sarah Thompson (Technical Writer, Programming Monthly). “Understanding how to effectively implement decorators is crucial for modern software development. They not only enhance functionality but also promote better testing practices by allowing for easier mocking and patching of functions during unit tests.”
Frequently Asked Questions (FAQs)
Can Decorator be used in Python?
Yes, Decorators can be used in Python to modify the behavior of functions or methods. They allow for the addition of functionality without altering the original code.
Can Decorator accept arguments?
Yes, Decorators can accept arguments. This is achieved by defining a wrapper function within the decorator that takes additional parameters, allowing for dynamic behavior based on the input.
Can Decorator be applied to classes?
Yes, Decorators can be applied to classes in Python. Class decorators can modify or enhance class definitions, similar to how function decorators work for functions.
Can Decorator be stacked?
Yes, Decorators can be stacked by applying multiple decorators to a single function or method. The decorators are applied in the order they are listed, allowing for layered functionality.
Can Decorator be used with built-in functions?
Yes, Decorators can be used with built-in functions. However, care should be taken to ensure that the decorator does not disrupt the expected behavior of the built-in function.
Can Decorator be removed after being applied?
No, once a Decorator is applied to a function or method, it cannot be removed in a straightforward manner. The original function is replaced by the decorated version, and the original reference is lost unless explicitly preserved.
The concept of “Can Decorator” revolves around the application of the decorator design pattern in programming, particularly in object-oriented languages. This pattern allows for the dynamic addition of behavior or responsibilities to individual objects without modifying the structure of the existing code. By using decorators, developers can enhance the functionality of classes in a flexible and reusable manner, promoting the principles of single responsibility and open/closed in software design.
One of the key takeaways from the discussion on Can Decorator is its ability to facilitate code maintenance and scalability. By employing decorators, developers can isolate changes to specific functionalities, reducing the risk of introducing bugs when modifying existing code. This modular approach not only streamlines the development process but also encourages cleaner code practices, making it easier for teams to collaborate and adapt to changing project requirements.
Additionally, the Can Decorator pattern exemplifies the importance of composition over inheritance in software design. While inheritance can lead to rigid class hierarchies, decorators allow for greater flexibility by enabling the combination of behaviors at runtime. This results in more versatile and adaptable code structures, which can be particularly beneficial in complex systems where functionality may need to evolve over time.
Author Profile
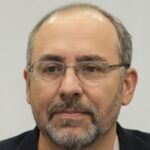
-
Mahlon Boehs is a seasoned entrepreneur and industry expert with a deep understanding of wood truss manufacturing and construction materials. As the President of Timberlake TrussWorks, LLC, Mahlon played a pivotal role in shaping the company’s reputation for quality and precision. His leadership ensured that each truss met rigorous structural standards, providing builders with dependable components essential to their projects.
Beginning in 2025, Mahlon Boehs has shifted his focus to education and knowledge-sharing through an informative blog dedicated to wood truss manufacturing. Drawing from his extensive experience in the field, he provides in-depth insights into truss design, material selection, and construction techniques. This blog serves as a valuable resource for builders, contractors, and homeowners seeking practical guidance on truss systems and structural integrity.
Latest entries
- March 18, 2025General Wood TopicsWhat Color Is Ebony Wood? Unveiling the Rich Hues of This Luxurious Timber
- March 18, 2025Construction & FrameworkHow Can You Effectively Mount a Headboard to Your Wall?
- March 18, 2025General Wood TopicsCan Bees Really Eat Wood? Unraveling the Myths and Facts
- March 18, 2025General Wood TopicsDo Rabbits Really Chew on Wood? Exploring Their Eating Habits!